Have you ever wondered how to protect our objects in JavaScript against any modification? Well if yes then you will be surprised to know that javascript has some predefined methods to prevent your objects from any modification and that methods are object seal vs object freeze.If you are confused about these techniques then dont worry you are at the right place. In this blog will dive deep into the history of these methods(you can also call techniques)and learn and compare object seal vs object freeze.
Welcome to the ultimate guide on object seal vs object freeze in JavaScript for web development! As a web developer, it is important to understand how to handle and manipulate JavaScript objects to create dynamic and interactive websites. In this comprehensive guide, we’ll take an in-depth look at the concepts of object seal and object freeze, exploring their differences, applications, and benefits in web development. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to make informed decisions when working with JavaScript objects.
Understanding Javascript And Objects
Before getting deep into the seal and Freeze our first step is to get the basic knowledge about what is javascript and what is an object. so first let’s have some basic knowledge about these.
What is Javascript?
JavaScript is a versatile programming language that plays a pivotal role in modern web development. When used judiciously, it can enhance user experiences, create dynamic content, and make websites more interactive. Let’s delve into its essence:
- Purpose: JavaScript allows you to implement complex features on web pages. Whenever a web page does more than just display static information, JavaScript is likely involved. It enables timely content updates, interactive maps, animated graphics, and more.
- Layer Cake: Imagine web technologies as a three-layer cake:
- HTML: The markup language that structures web content (e.g., paragraphs, headings, images).
- CSS: The language for styling HTML content (e.g., colors, fonts, layout).
- JavaScript: The scripting language that brings dynamic behavior to web pages.
What is Object?
- An object in JavaScript is a non-primitive data type used to store multiple values. These values are organized as key-value pairs.
- Think of an object like a container that holds related information. Each key represents a property, and its associated value can be of any data type (string, number, boolean, or even another object).
The key features of objects in JavaScript include:
- Properties: Objects can have properties, which are key-value pairs that hold data.
- Methods: Objects can have methods, which are functions associated with the object’s behavior.
- Dynamic: Objects in JavaScript are dynamic, meaning you can add or remove properties and methods at any time.
- Prototype-based: JavaScript objects inherit properties and methods from a prototype, allowing for easy sharing of functionality.
- Access Control: Properties and methods can be marked as writable, enumerable, or configurable to control their accessibility and behavior.
- Serialization: Objects can be converted into strings using serialization methods like
JSON.stringify()
for data interchange. - Iterability: Objects can be iterated over using loops or iteration methods like
for...in
loop orObject.keys()
.
These features make objects versatile and powerful constructs in JavaScript, allowing for flexible data modeling and manipulation.
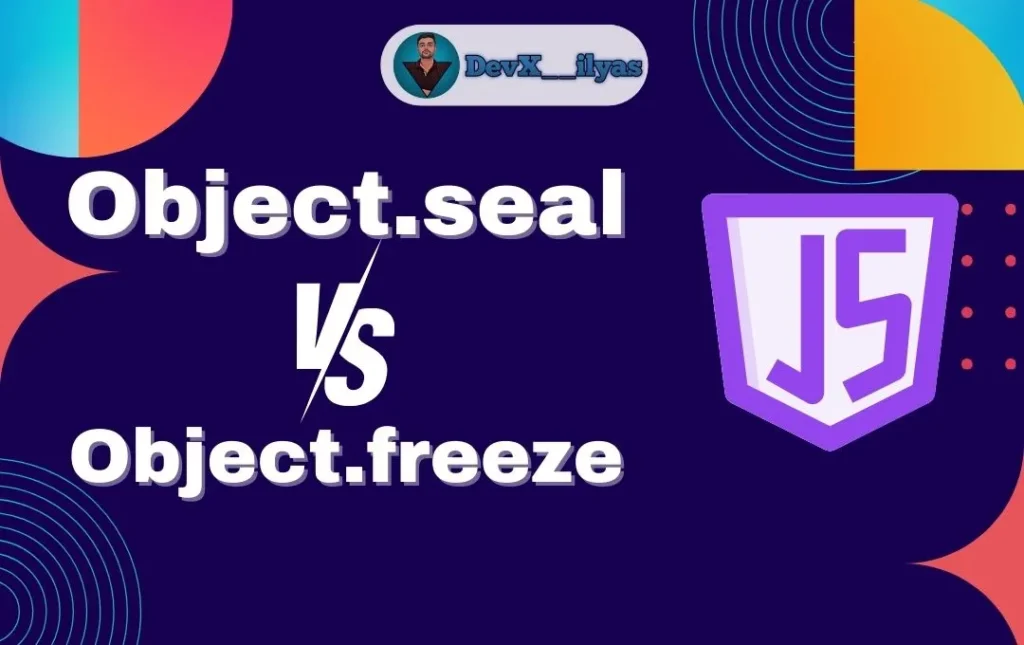
Object Seal: Securing with Limited Flexibility
Object sealing is a technique in JavaScript to mark an object as sealed, thereby preventing the addition of new properties and reconfiguration of existing ones after sealing. However, object seals do not affect the ability to modify or delete existing properties.
Syntax and Usage
To seal an object in JavaScript, you can use the Object.seal()
method. Let’s take a look at the syntax:
Object.seal(object);
The Object.seal()
method can be applied to any object, from simple ones to deeply nested complex structures. Once an object is sealed, it cannot be unsealed.
Characteristics and Limitations
Object sealing offers a level of protection, making it useful in scenarios where you want to restrict changes to an object’s structure while still allowing modifications to the existing properties. Here are some key characteristics and limitations of object seals:
- Prevents property addition: After an object is sealed, you cannot add new properties to it. Any attempt to add a new property will be ignored.
- Prevents property deletion: Object seals do not allow the deletion of existing properties. Once sealed, properties cannot be removed.
- Allows property modification: It is still possible to modify the existing properties within a sealed object. Values and attributes can be changed, but the structure remains intact.
- Retains inheritance and descriptors: Object seals preserve the inheritance and descriptor attributes of properties.
- Scoped to direct properties: Sealing an object does not automatically seal its nested objects or properties. Only the direct properties are sealed.
Callout: Object sealing strikes a balance between protecting an object’s structure and allowing necessary modifications to its properties. It’s a valuable tool in scenarios where you want control without complete immutability.
Use Cases for Object Sealing
Object sealing can be particularly handy in certain situations where the structure of an object needs to be maintained with limited flexibility. Here are a few notable use cases:
- Data validation: When handling user input, an object seal can help ensure that only a specific set of properties are present, reducing the risk of unexpected or inconsistent data.
- Configuration objects: Object sealing proves useful in configurations, enabling developers to prevent any accidental additions or removals of properties, while still allowing customization within the object.
- Security: By sealing designated objects, you can safeguard sensitive information from being tampered with while still allowing modifications to non-sensitive properties.
Note: External resources like MDN web docs can further enhance your understanding of object sealing.
More popular post from DevXilyas
- Leadpages Vs Clickfunnels – The Ultimate Guide
- Best Node Js Microservices Framework To Use In 2022
- What Is Bespoke Software ? The Ultimate Guide
- Best React Design Patterns You Must Know In 2023
- Best React UI Frameworks In 2023
Object Freeze: Immutability at Its Core
Unlike object sealing, object freezing takes immutability to the next level. When an object is frozen, it becomes completely unmodifiable, protecting not only the structure but also the values and attributes of properties.
Syntax and Usage
JavaScript provides the Object.freeze()
method to freeze an object. The syntax is as follows:
Object.freeze(object);
The Object.freeze()
method ensures that any attempt to modify, delete, or add properties to the object will be strictly ignored.
Characteristics and Limitations
By freezing an object, you achieve a higher level of immutability with full protection against modifications. Consider the following characteristics and limitations of object freezing:
- Prevents property addition: Freezing an object not only disallows adding new properties but also ignores any attempts to modify existing properties.
- Prevents property deletion: Just like sealing, object freezing prohibits the deletion of properties. Once frozen, no properties can be removed.
- Prevents property modification: Object freezing goes beyond sealing by also disallowing the modification of existing properties. Values and attributes become read-only.
- Preserves inheritance and descriptors: Similar to object sealing, object freezing maintains inheritance and property descriptor attributes.
- Freezes nested objects: When an object is frozen, any objects nested within it are also recursively frozen.
Callout: Object freezing provides the highest level of immutability, making it perfect for situations where you want complete structural integrity and data immutability.
Use Cases for Object Freezing
As one might expect, object freezing finds its greatest utility in scenarios that demand an immutable nature. Let’s explore a few prominent use cases:
- Constants or configurations: Using object freezing is an excellent approach when you want to define constants or configurations that should remain unchanged throughout the execution of a program.
- Shared objects: In scenarios where multiple components or threads need access to the same object, freezing it ensures consistency and prevents unexpected modifications.
- Caching and memoization: Object freezing is valuable in caching or memoization scenarios, where the integrity of cached results must be preserved to prevent unexpected errors or inconsistencies.
Note: External resources like MDN web docs can provide additional insights into object freezing.
Use case examples of Object Seal vs Object freeze
Now ,till now you have learned all about Object Seal vs Object freeze.its time to see some use case examples of Object Seal and Object freeze.
Object.seal()
:
Object.seal(obj)
prevents adding or removing properties from the sealed object.- Existing properties become non-configurable (cannot be converted from data descriptors to accessor descriptors).
- Attempting to modify the value of the sealed object itself can throw a
TypeError
(especially in strict mode). - Example:
const person = { name: "Alice", age: 30 }; Object.seal(person); // Now let's try to modify properties person.age = 31; // Allowed person.city = "New York"; // Not allowed (no new properties) delete person.name; // Not allowed (cannot remove properties) console.log(person); // { name: 'Alice', age: 31 }
Object.freeze()
:
Object.freeze(obj)
does everythingObject.seal()
does, plus it prevents modifying any existing properties.- Properties become both non-configurable and non-writable.
- Example:
const config = { appName: "MyApp", version: "1.0" }; Object.freeze(config); // Now let's try to modify properties config.version = "2.0"; // Not allowed (properties are read-only) config.newFeature = true; // Not allowed (no new properties) console.log(config); // { appName: 'MyApp', version: '1.0' }